What ComputerCraft is about?
It is a mod you can download and install
- Mods (short for modifications) are anything that changes Minecraft’s game content from what it originally was.
ComputerCraft adds computers that you can program in Lua
- Lua is a powerful, efficient, lightweight, embeddable scripting language.
- “Lua” (pronounced LOO-ah) means “Moon” in Portuguese. As such, it is neither an acronym nor an abbreviation, but a noun.
ComputerCraft also adds Turtles – little robots
How to craft a computer?
Recipe for a computer:
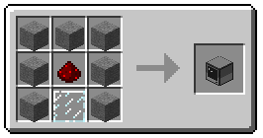
Advanced computer (mouse support & colors):
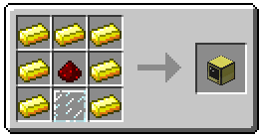
This is what your computer should look like
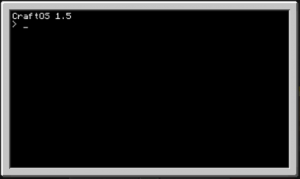
This is called the terminal or command line interface.
We can type into this and the computer will receive the keystrokes.
Commands to practice
list
The list program shows the files and folders in the current directory
list <name>
We can see the contents of the <name> folder
cd <name>
Change our current directory to the <name> folder
cd ..
Changes the directory to be one level higher than the current directory
lua
The “lua” program starts another command-line interface where we can run lua code directly
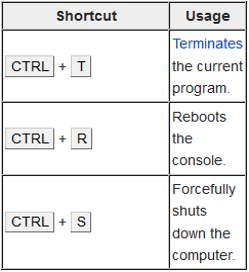
Let’s run little bits of actual lua code
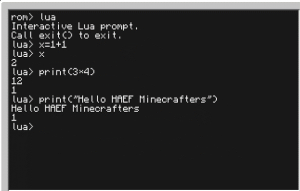
Type in ‘x = 1 + 1’ and hit Enter
Type ‘x’
Type ‘print(“hello world”)’
exit() function to exit the lua prompt
… and that’s it!
Create/edit a file
edit startup
Tells the edit program to edit the file named “startup” in the current directory. Since that file doesn’t exist, it will open up a blank editor.
When we save the file in the editor, it will automatically create the file since it did not exist already.
The edit program is one of the programs in ComputerCraft that requires an argument when you start it.
edit myFile
Type ‘print(“Hello minecrafters”)’
Ctrl > Save > Exit
Type ‘myFile’
How to print, do math, run a program
How to print stuff to a computer
print()
print(“Hello”)
How to do math with a computer
print(5+5)
How to run a program
Just type the name of the file/program
Declare Variables
local variables
local a=10
print(a^2)
Get input from user
print(“type your name”)
Declare the variable name to store the answer of the user
local name=read()
print(name)
print(“name”)
The “if” statement
The general form of the if statement is the following:
if <condition> then
<commands>
else
<commands>
end
Create a program to ask your name, read it and if it is valid print “Hello”, if not, print “Who are you?”
if (name==“Christos”) then
print (“Hello”)
else
print (“Who are you?”)
end
Craft a disk drive and a disk
Recipe for disk drive (device needed to read floppy disks):
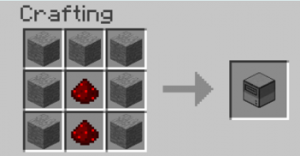
Floppy disk (a storage media to copy files):
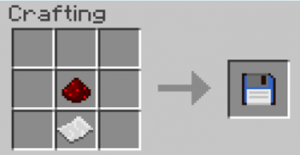
Use a floppy disk
Place the disk drive directly adjacent to the computer
Right click the disk drive to open its inventory interface and place the floppy disk into the drive
If you attach successfully a disk drive to a computer, by typing “list” you see that there is a folder named “disk”.
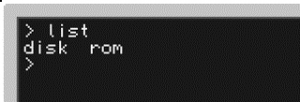
You can now create, edit, move or copy files into the disk and exchange files with other players or computers.
Assign a label to a disk
You can assign the disk a label
Use the same label command you used to set the computer’s label
You have to include the name of the side that the disk drive is on, in order to set the disk’s label rather than the computer’s.
If your disk drive is on the right side of the computer, you should use the command `label set right test-disk` to set the disk’s label to “test-disk“
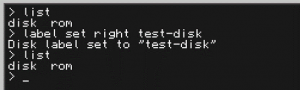
Exercise 1: Create a program in a disk
This program should:
- Ask for your name
- While the name you type is not the correct one, ask again
- The loop should end when you type the correct name (your name)
Solution
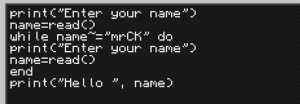
Reviewing the if statement
The if statement is a Control Structure
Control structures effect the flow of the program
The if statement is executed once
if <condition> then
<commands>
else
<commands>
end
Example of an if statement
x = 1
print(“Let’s test if x > 0 …”)
if (x > 0) then
print(“It is”)
else
print(“It is not”)
end
print(“Test is over”)
— Result:
— Let’s test if x > 0 …
— It is.
— Test is over
Let’s explain the above code:
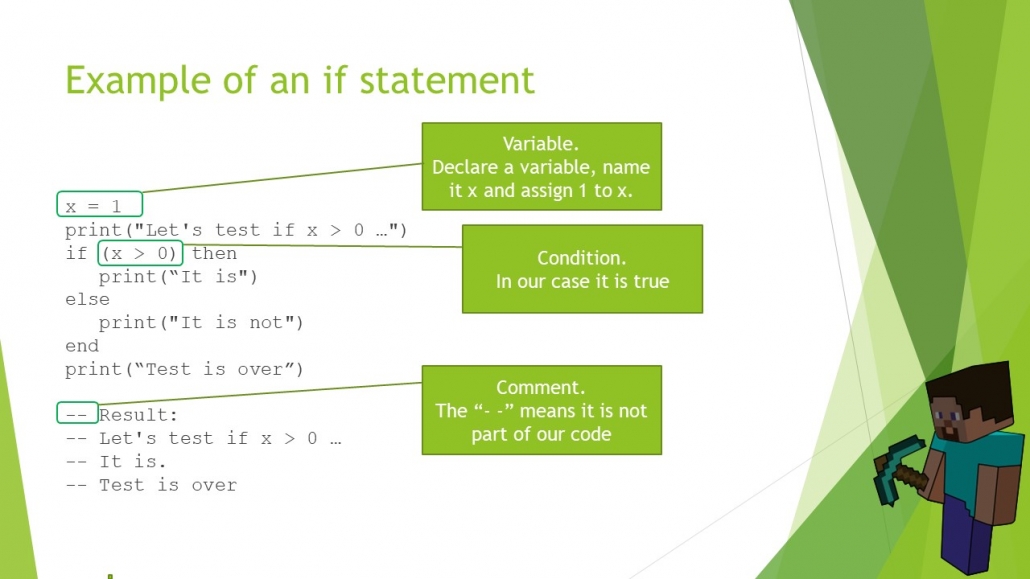
The while loop
The while statement is a control function
It keeps running until the condition is false
A condition could be either true or false
while <condition> do
<commands>
end
Example of a while loop
–Example of while control structure
x = 1
print(“Let’s count up to 5“)
while x <= 5 do
print(x,“…”)
x = x + 1
end
— result:
— Let’s count up to 5
— 1…
— 2…
— 3…
— 4…
— 5…
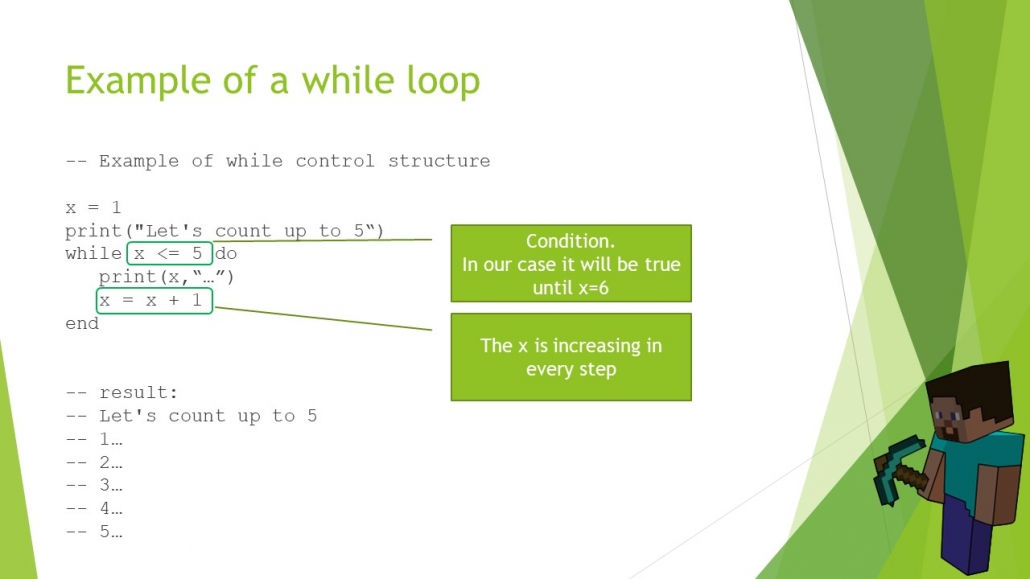
Exercise 2: Create a password protected door
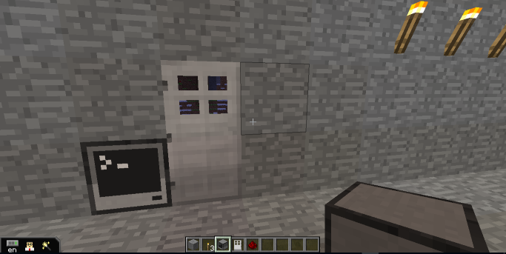
Create an iron door
Place a computer next to it or connect the computer to the door using redstone
Create a program in the computer and name it startup (to run every time the computer reboots)
The program must ask for the password (“haef”) and grant access or not
Solution
print(“Give the password”)
a = read()
if a == “minecraft” then
print(“Access granted”)
redstone.setOutput(“right”,true)
sleep(5)
redstone.setOutput(“right”,false)
else
print(“Access denied”)
sleep(2)
end
reboot()
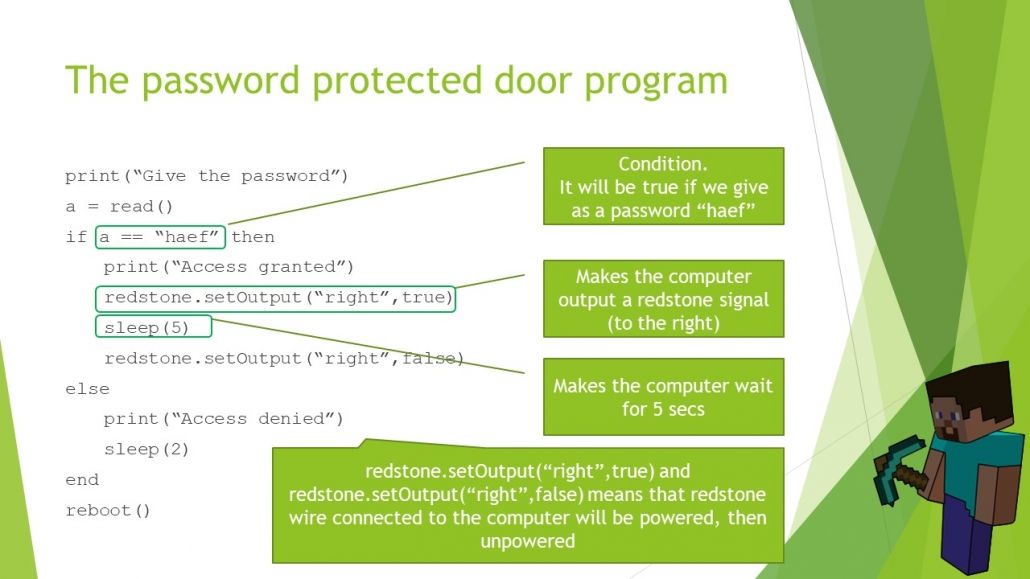