Variables
In this lesson, we are introduced to the concept of a variable.
A variable is a location in a computer’s memory where we can hold information used by our program – just like storing things in a box.
Each storage location in memory (i.e. variable) has three features:
i. Name
The name of a variable must be unique inside the program. The variable is identified by its name. We can use whatever name we like, but there are conventions we must follow.
We should always give a variable a sensible name that tells us what kind of information is stored in it – just like putting a label on the box to tell us what’s inside. For example if we wnt to store in a variable a price, we should name the particular variable “price”. There is no difference for the computer, but it makes a huge difference for the person who reads, or develops the program.
ii. Type
Each variable has a data type, depending on the type of the data we need to store inside the variable. Most programming languages have the integer type (for whole numbers), the float type (for real numbers), the boolean type (for boolean values, i.e. true or false), the character type (for a single character, letter or digit) and the string type (for alphanumerical strings, letters and/or digits).
We may need to declare the variables prior to assign a value, or not. It depends on the language. In C++ or Java we must declare in advance the type and the name of the variable, in Python we can use directly the variable.
iii. Value
The value of a variable is its content. We may assign a value to a variable (e.g. “a=1” in Python). Or, we may input a value from the user and use the variable to store it (e.g. “cin >> a;” in C++).
Once a variable is created, the information stored inside it can be set or changed (that is, varied – hence the word “variable”).
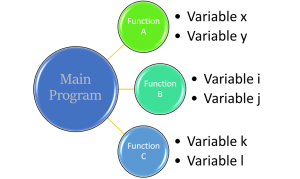
Variables x and y are recognizable only in Function A.
Variables i and j are recognizable only in Function B.
Variables k and l are recognizable only in Function C.
The scope of a variable
Apart from the three features, a variable has a scope. The scope defines the part of the program where the particular variable is available to the user. By default a variable’s scope is defined by the place where the variable is created. The variable is not recognizable outside this part (we usually call this part function).
Have a look at the following code in Python.
We defined a function named max() which accepts two variables (a, b) and assign their values in variables x, y. Variables x and y are only recognizable inside the function max().
We usually call a fubction’s variables local. Their scope is limitied inside the function.
On the other hand, we may use global variables. They are recognizable in all the functions of a program.
#define function with def def max(x, y): if x>y: return x else: return y a = int(input ('Enter a number\n')) b = int(input ('Enter another number\n')) print('Output the max number between %s and %s' %(a,b)) #call function max with parameters print ('max is', max(a,b))